Note
Go to the end to download the full example code.
Spatial Correlation Coefficient¶
The Spatial Correlation Coefficient can be applied to compare the spatial structure of two images, which can be valuable in various domains such as medical imaging, remote sensing, and quality assessment in manufacturing or design processes.
Let’s consider a use case in medical imaging where Spatial Correlation Coefficient is used to compare the spatial correlation between a reference image and a reconstructed medical scan. This can be particularly relevant in evaluating the accuracy of image reconstruction techniques or assessing the quality of medical imaging data.
Here’s a hypothetical Python example demonstrating the usage of the Spatial Correlation Coefficient to compare two medical images:
14 import matplotlib.pyplot as plt
15 import numpy as np
16 import torch
17 from skimage.data import shepp_logan_phantom
18 from skimage.transform import iradon, radon, rescale
19 from torchmetrics.image import SpatialCorrelationCoefficient
Create a Shepp-Logan phantom image
Simulate projection data (sinogram) using Radon transform
28 theta = np.linspace(0.0, 180.0, max(phantom.shape), endpoint=False)
29 sinogram = radon(phantom, theta=theta)
Perform reconstruction using the inverse Radon transform
33 reconstruction = iradon(sinogram, theta=theta, circle=True)
Display the results
37 fig, (ax1, ax2, ax3) = plt.subplots(1, 3, figsize=(10, 4))
38 ax1.set_title("Original")
39 ax1.imshow(phantom, cmap=plt.cm.Greys_r)
40 ax2.set_title("Radon transform (Sinogram)")
41 ax2.imshow(sinogram, cmap=plt.cm.Greys_r, extent=(0, 180, 0, sinogram.shape[0]), aspect="equal")
42 ax3.set_title("Reconstruction from sinogram")
43 ax3.imshow(reconstruction, cmap=plt.cm.Greys_r)
44 fig.tight_layout()
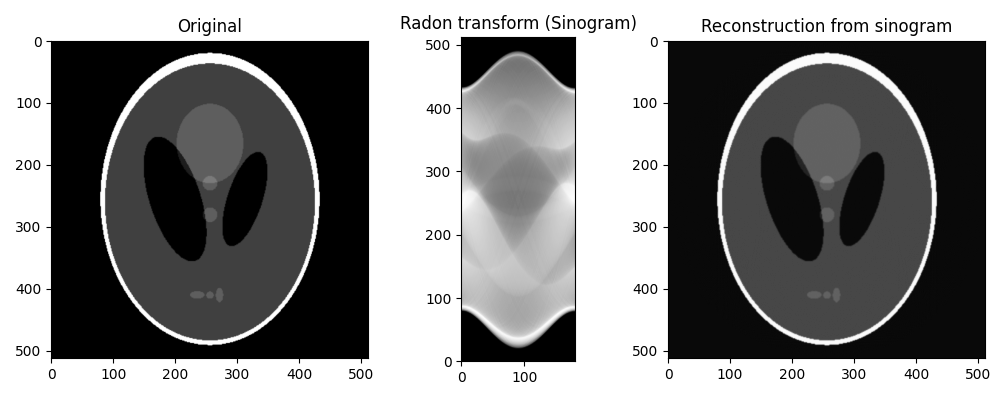
Convert the images to PyTorch tensors
48 phantom_tensor = torch.from_numpy(phantom).float().unsqueeze(0).unsqueeze(0)
49 reconstructed_tensor = torch.from_numpy(reconstruction).float().unsqueeze(0).unsqueeze(0)
Calculating the Spatial Correlation Coefficient
53 scc = SpatialCorrelationCoefficient()
54 score = scc(preds=reconstructed_tensor, target=phantom_tensor)
55
56 print(f"Spatial Correlation Coefficient between the images: {score}")
57 fig.suptitle(f"Spatial Correlation Coefficient: {score:.5}", y=-0.01)
Spatial Correlation Coefficient between the images: 0.14591339230537415
Text(0.5, -0.01, 'Spatial Correlation Coefficient: 0.14591')
Total running time of the script: (0 minutes 3.200 seconds)