Note
Go to the end to download the full example code.
Signal-to-Noise Ratio¶
Imagine developing a song recognition application. The software’s goal is to recognize a song even when it’s played in a noisy environment, similar to Shazam. To achieve this, you want to enhance the audio quality by reducing the noise and evaluating the improvement using the Signal-to-Noise Ratio (SNR).
In this example, we will demonstrate how to generate a clean signal, add varying levels of noise to simulate the noisy recording, use FFT for noise reduction, and then evaluate the quality of the reconstructed audio using SNR.
Import necessary libraries
12 import matplotlib.animation as animation
13 import matplotlib.pyplot as plt
14 import numpy as np
15 import torch
16 from torchmetrics.audio import SignalNoiseRatio
Generate a clean signal (simulating a high-quality recording)
22 def generate_clean_signal(length: int = 1000) -> tuple[np.ndarray, np.ndarray]:
23 """Generate a clean signal (sine wave)"""
24 t = np.linspace(0, 1, length)
25 signal = np.sin(2 * np.pi * 10 * t) # 10 Hz sine wave, representing the clean recording
26 return t, signal
Add Gaussian noise to the signal to simulate the noisy environment
33 def add_noise(signal: np.ndarray, noise_level: float = 0.5) -> np.ndarray:
34 """Add Gaussian noise to the signal."""
35 noise = noise_level * np.random.randn(signal.shape[0])
36 return signal + noise
Apply FFT to filter out the noise
43 def fft_denoise(noisy_signal: np.ndarray, threshold: float) -> np.ndarray:
44 """Denoise the signal using FFT."""
45 freq_domain = np.fft.fft(noisy_signal) # Filter frequencies using FFT
46 magnitude = np.abs(freq_domain)
47 filtered_freq_domain = freq_domain * (magnitude > threshold)
48 return np.fft.ifft(filtered_freq_domain).real # Perform inverse FFT to reconstruct the signal
Generate and plot clean, noisy, and denoised signals to visualize the reconstruction
54 length = 1000
55 t, clean_signal = generate_clean_signal(length)
56 noisy_signal = add_noise(clean_signal, noise_level=0.5)
57 denoised_signal = fft_denoise(noisy_signal, threshold=10)
58
59 plt.figure(figsize=(12, 4))
60 plt.plot(t, noisy_signal, label="Noisy environment", color="blue", alpha=0.7)
61 plt.plot(t, denoised_signal, label="Denoised signal", color="green", alpha=0.7)
62 plt.plot(t, clean_signal, label="Clean song", color="red", linewidth=3)
63 plt.xlabel("Time")
64 plt.ylabel("Amplitude")
65 plt.title("Clean Song vs. Noisy Environment vs. Denoised Signal")
66 plt.legend()
67 plt.show()
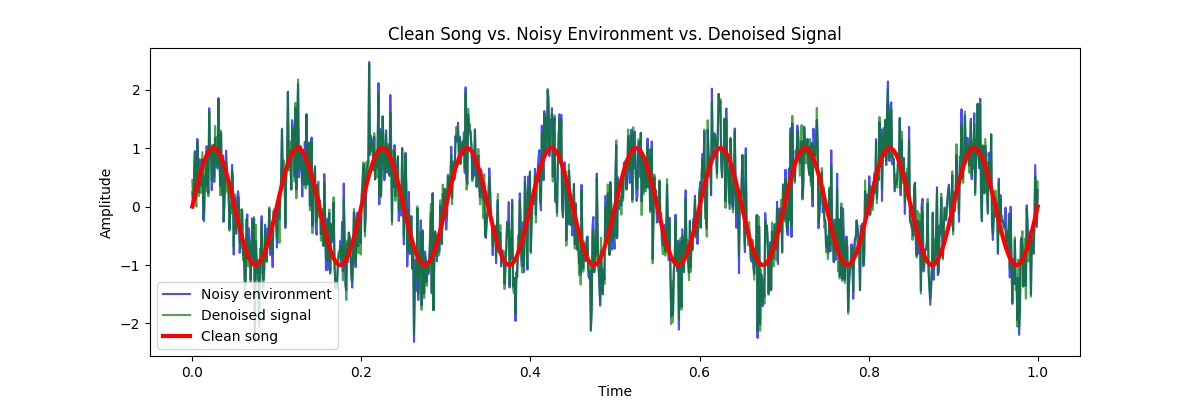
Convert the signals to PyTorch tensors and calculate the SNR
71 clean_signal_tensor = torch.tensor(clean_signal).float()
72 noisy_signal_tensor = torch.tensor(noisy_signal).float()
73 denoised_signal_tensor = torch.tensor(denoised_signal).float()
74
75 snr = SignalNoiseRatio()
76 initial_snr = snr(preds=noisy_signal_tensor, target=clean_signal_tensor)
77 reconstructed_snr = snr(preds=denoised_signal_tensor, target=clean_signal_tensor)
78 print(f"Initial SNR: {initial_snr:.2f}")
79 print(f"Reconstructed SNR: {reconstructed_snr:.2f}")
Initial SNR: 3.48
Reconstructed SNR: 3.85
To show the effect of different noise levels on the SNR, we create an animation that iterates over different noise levels and updates the plot accordingly:
83 fig, ax = plt.subplots(figsize=(12, 4))
84 noise_levels = [0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9]
85
86
87 def update(num: int) -> tuple:
88 """Update the plot for each frame."""
89 t, clean_signal = generate_clean_signal(length)
90 noisy_signal = add_noise(clean_signal, noise_level=noise_levels[num])
91 denoised_signal = fft_denoise(noisy_signal, threshold=10)
92
93 clean_signal_tensor = torch.tensor(clean_signal).float()
94 noisy_signal_tensor = torch.tensor(noisy_signal).float()
95 denoised_signal_tensor = torch.tensor(denoised_signal).float()
96 initial_snr = snr(preds=noisy_signal_tensor, target=clean_signal_tensor)
97 reconstructed_snr = snr(preds=denoised_signal_tensor, target=clean_signal_tensor)
98
99 ax.clear()
100 (noisy,) = plt.plot(t, noisy_signal, label="Noisy Environment", color="blue", alpha=0.7)
101 (denoised,) = plt.plot(t, denoised_signal, label="Denoised Signal", color="green", alpha=0.7)
102 (clean,) = plt.plot(t, clean_signal, label="Clean Song", color="red", linewidth=3)
103 ax.set_xlabel("Time")
104 ax.set_ylabel("Amplitude")
105 ax.set_title(
106 f"Initial SNR: {initial_snr:.2f} - Reconstructed SNR: {reconstructed_snr:.2f} - Noise level: {noise_levels[num]}"
107 )
108 ax.legend(loc="upper right")
109 ax.set_ylim(-3, 3)
110 return noisy, denoised, clean
111
112
113 ani = animation.FuncAnimation(fig, update, frames=len(noise_levels), interval=1000)
Total running time of the script: (0 minutes 3.185 seconds)