Note
Go to the end to download the full example code.
Evaluating Speech Quality with PESQ metric¶
- This notebook will guide you through calculating the Perceptual Evaluation of Speech Quality (PESQ) score,
a key metric in assessing how effective noise reduction and enhancement techniques are in improving speech quality. PESQ is widely adopted in industries such as telecommunications, VoIP, and audio processing. It provides an objective way to measure the perceived quality of speech signals from a human listener’s perspective.
- Imagine being on a noisy street, trying to have a phone call. The technology behind the scenes aims
to clean up your voice and make it sound clearer on the other end. But how do engineers measure that improvement? This is where PESQ comes in. In this notebook, we will simulate a similar scenario, applying a simple noise reduction technique and using the PESQ score to evaluate how much the speech quality improves.
Import necessary libraries
18 import matplotlib.pyplot as plt
19 import numpy as np
20 import torch
21 import torchaudio
22 from torchmetrics.audio import PerceptualEvaluationSpeechQuality
Generate Synthetic Clean and Noisy Audio Signals We’ll generate a clean sine wave (representing a clean speech signal) and add white noise to simulate the noisy version.
29 def generate_sine_wave(frequency, duration, sample_rate, amplitude: float = 0.5):
30 """Generate a clean sine wave at a given frequency."""
31 t = torch.linspace(0, duration, int(sample_rate * duration))
32 return amplitude * torch.sin(2 * np.pi * frequency * t)
33
34
35 def add_noise(waveform: torch.Tensor, noise_factor: float = 0.05) -> torch.Tensor:
36 """Add white noise to a waveform."""
37 noise = noise_factor * torch.randn(waveform.size())
38 return waveform + noise
39
40
41 # Parameters for the synthetic audio
42 sample_rate = 16000 # 16 kHz typical for speech
43 duration = 3 # 3 seconds of audio
44 frequency = 440 # A4 note, can represent a simple speech-like tone
45
46 # Generate the clean sine wave
47 clean_waveform = generate_sine_wave(frequency, duration, sample_rate)
48
49 # Generate the noisy waveform by adding white noise
50 noisy_waveform = add_noise(clean_waveform)
Apply Basic Noise Reduction Technique In this step, we apply a simple spectral gating method for noise reduction using torchaudio’s spectrogram method. This is to simulate the enhancement of noisy speech.
59 def reduce_noise(noisy_signal: torch.Tensor, threshold: float = 0.2) -> torch.Tensor:
60 """Basic noise reduction using spectral gating."""
61 # Compute the spectrogram
62 spec = torchaudio.transforms.Spectrogram()(noisy_signal)
63
64 # Apply threshold-based gating: values below the threshold will be zeroed out
65 spec_denoised = spec * (spec > threshold)
66
67 # Convert back to the waveform
68 return torchaudio.transforms.GriffinLim()(spec_denoised)
69
70
71 # Apply noise reduction to the noisy waveform
72 enhanced_waveform = reduce_noise(noisy_waveform)
Initialize the PESQ Metric PESQ can be computed in two modes: ‘wb’ (wideband) or ‘nb’ (narrowband). Here, we are using ‘wb’ mode for wideband speech quality evaluation.
78 pesq_metric = PerceptualEvaluationSpeechQuality(fs=sample_rate, mode="wb")
Compute PESQ Scores We will calculate the PESQ scores for both the noisy and enhanced versions compared to the clean signal. The PESQ scores give us a numerical evaluation of how well the enhanced speech compares to the clean speech. Higher scores indicate better quality.
86 pesq_noisy = pesq_metric(clean_waveform, noisy_waveform)
87 pesq_enhanced = pesq_metric(clean_waveform, enhanced_waveform)
88
89 print(f"PESQ Score for Noisy Audio: {pesq_noisy.item():.4f}")
90 print(f"PESQ Score for Enhanced Audio: {pesq_enhanced.item():.4f}")
PESQ Score for Noisy Audio: 3.0618
PESQ Score for Enhanced Audio: 1.3961
Visualize the waveforms We can visualize the waveforms of the clean, noisy, and enhanced audio to see the differences.
95 fig, axs = plt.subplots(3, 1, figsize=(12, 9))
96
97 # Plot clean waveform
98 axs[0].plot(clean_waveform.numpy())
99 axs[0].set_title("Clean Audio Waveform (Sine Wave)")
100 axs[0].set_xlabel("Time")
101 axs[0].set_ylabel("Amplitude")
102
103 # Plot noisy waveform
104 axs[1].plot(noisy_waveform.numpy(), color="orange")
105 axs[1].set_title(f"Noisy Audio Waveform (PESQ: {pesq_noisy.item():.4f})")
106 axs[1].set_xlabel("Time")
107 axs[1].set_ylabel("Amplitude")
108
109 # Plot enhanced waveform
110 axs[2].plot(enhanced_waveform.numpy(), color="green")
111 axs[2].set_title(f"Enhanced Audio Waveform (PESQ: {pesq_enhanced.item():.4f})")
112 axs[2].set_xlabel("Time")
113 axs[2].set_ylabel("Amplitude")
114
115 # Adjust layout for better visualization
116 fig.tight_layout()
117 plt.show()
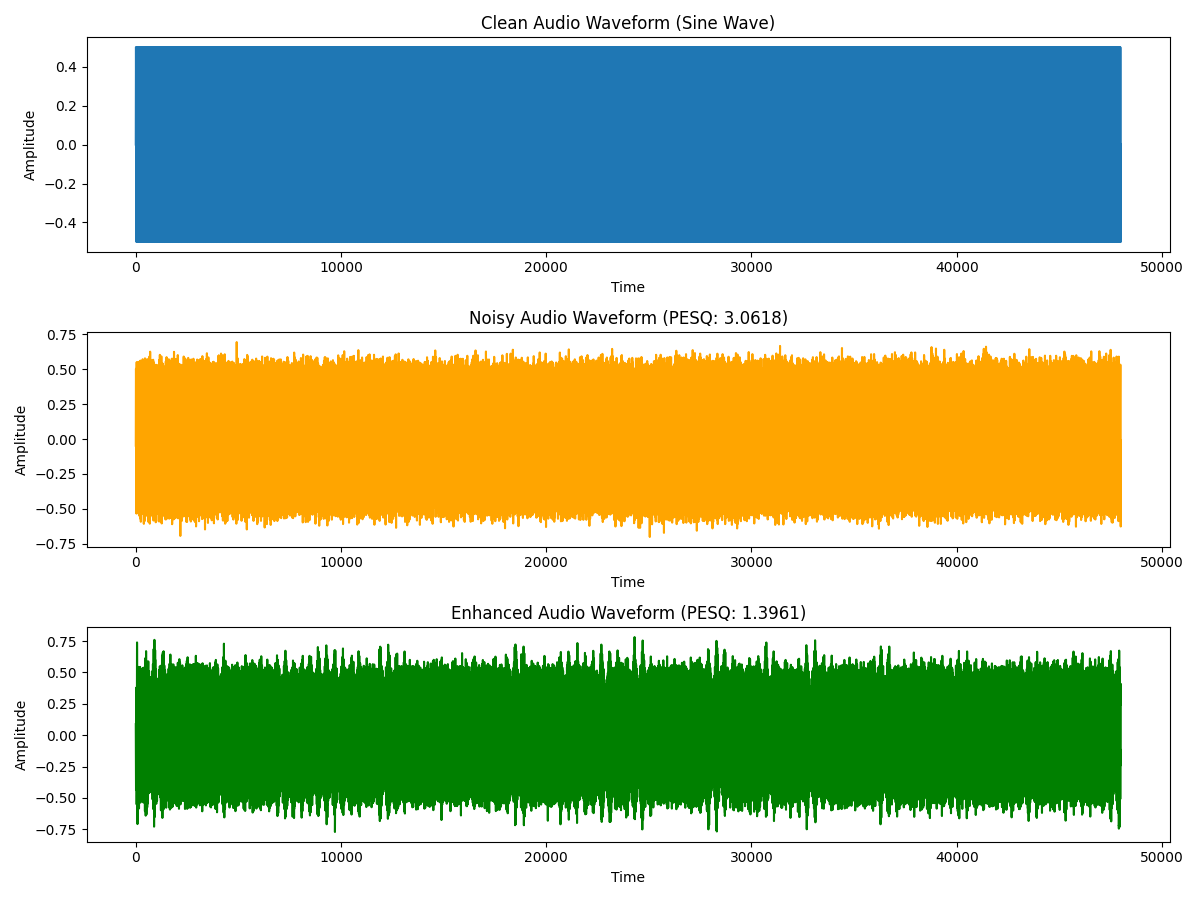
Total running time of the script: (0 minutes 0.681 seconds)